This blog shares how to create your own serverless ChatGPT API in Go.
What is Chat GPT ?
A natural language processing (NLP) model called GPT-3, also known as the "Generative Pre-training Transformer 3," was developed using text that humans produced. It can create its own human-like text using a range of languages and writing styles given some text input.
More details on Chat GPT and the excellent work can be found here.
What is Go?
Go, also referred to as Golang, is an open-source, statically typed, compiled computer language created by Google. It is designed to be straightforward, powerful, readable, and effective.
Prerequisites
The following prerequisites are needed -
- OpenAI Account - can be created here
- SAM CLI Installed - can be found here
- AWS Account(if you plan on deploying it to AWS)
Create Serverless API
- Run sam init
- Select AWS Quick Start Templates below
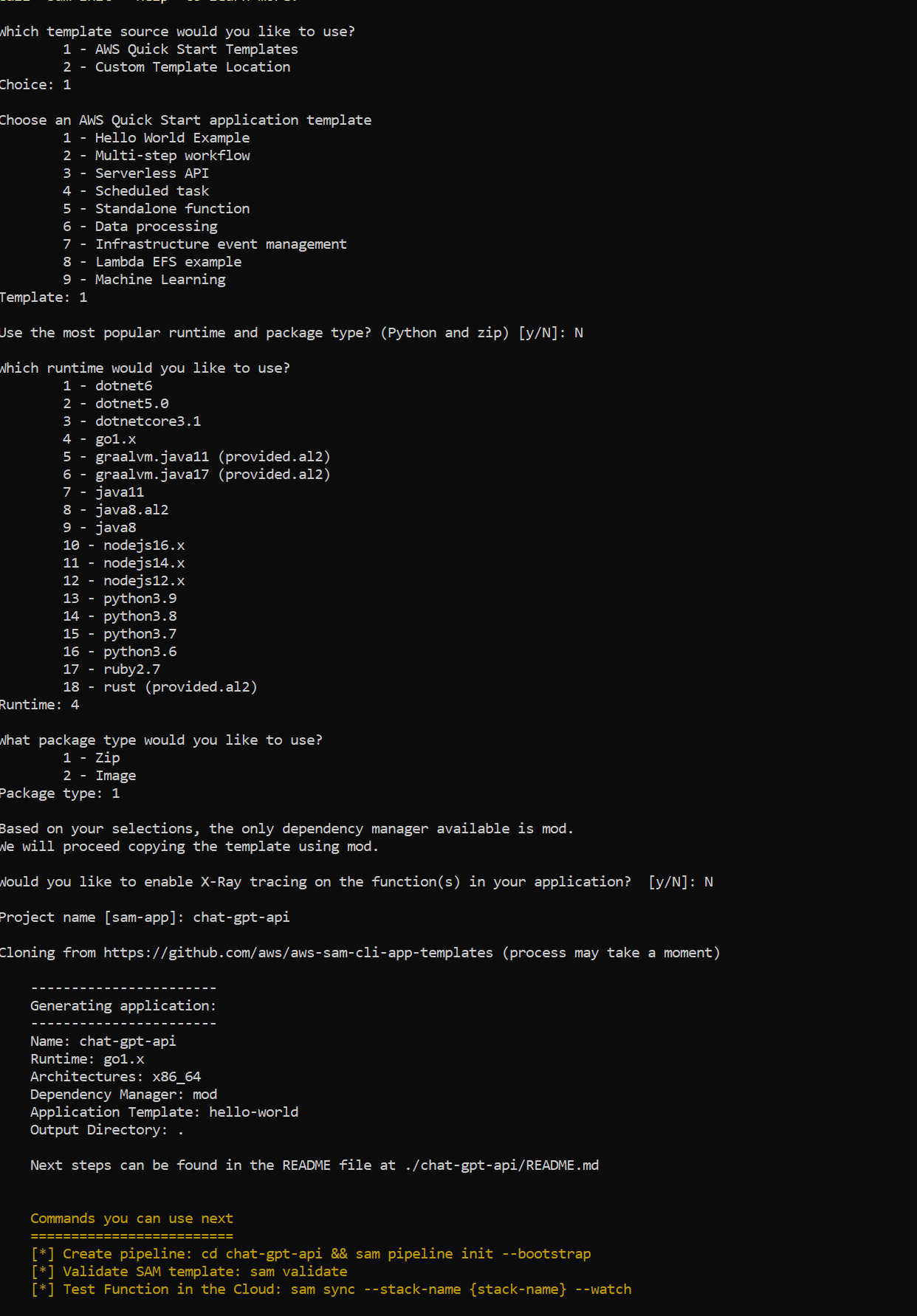
- Edit the structure to create a folder called pkg and add a main.go
- Create the functionality to call the Chat GPT API and return the response
- Create a struct TextCompletionRequest like the below which matches the format, Chat GPT API accepts
type TextCompletionRequest struct {
Model string `json:"model"`
Prompt string `json:"prompt"`
Temperature float64 `json:"temperature"`
MaxTokens int `json:"max_tokens"`
TopP float64 `json:"top_p"`
FrequencyPenalty float64 `json:"frequency_penalty"`
PresencePenalty float64 `json:"presence_penalty"`
}
- Create a struct TextCompletionResponse like the below which matches the format, Chat GPT API accepts
type Choice struct {
Text string `json:"text"`
Index int `json:"index"`
Logprobs interface{} `json:"logprobs"`
FinishReason string `json:"finish_reason"`
}
type TextCompletionResponse struct {
ID string `json:"id"`
Object string `json:"object"`
Created int `json:"created"`
Model string `json:"model"`
Choices []Choice `json:"choices"`
Usage struct {
PromptTokens int `json:"prompt_tokens"`
CompletionTokens int `json:"completion_tokens"`
TotalTokens int `json:"total_tokens"`
} `json:"usage"`
}
- Create function ConverseWithGPT function below
func ConverseWithGPT(prompt string) (TextCompletionResponse, error) {
httpReq := TextCompletionRequest{
Model: "text-davinci-003",
Prompt: prompt,
Temperature: 0.7,
MaxTokens: 100,
TopP: 1.0,
FrequencyPenalty: 0.0,
PresencePenalty: 0.0,
}
jsonValue, _ := json.Marshal(httpReq)
bearer := "Bearer " + BearerToken
req, _ := http.NewRequest("POST", ChatGPTHTTPAddress, bytes.NewBuffer(jsonValue))
req.Header.Set("Authorization", bearer)
req.Header.Add("Content-Type", "application/json")
client := &http.Client{}
resp, err := client.Do(req)
if err != nil {
return TextCompletionResponse{}, err
}
defer resp.Body.Close()
if resp.StatusCode != 200 {
return TextCompletionResponse{}, ErrNon200Response
}
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
return TextCompletionResponse{}, err
}
var textCompletionResponse TextCompletionResponse
if err := json.Unmarshal(body, &textCompletionResponse); err != nil {
fmt.Println("Can not unmarshal JSON")
}
return textCompletionResponse, nil
}
To check, the code, checkout this repo.
Let's see it in action
Let's now run this locally.
Run the below command -
sam build
sam local start-api
Now, we can run this using Postman
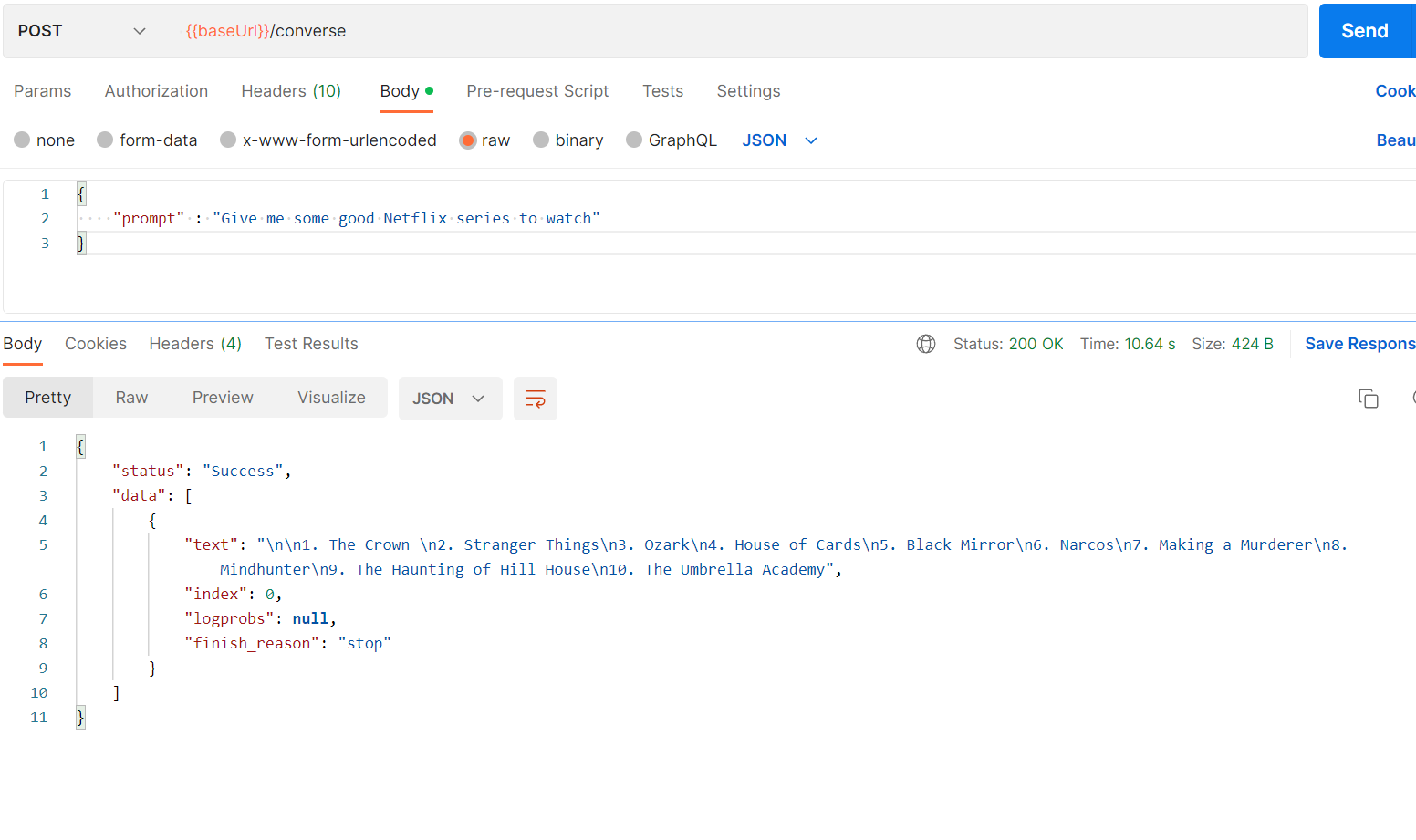
All right, Chat GPT suggested some Netflix series to watch. Let me go watch them while you try running this yourself.
Cheers 🍻